A Collection is identified by an ID. More...
#include <TrackerHitCollection.h>
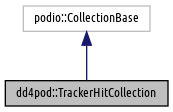
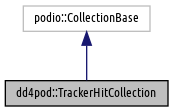
Public Types | |
using | const_iterator = const TrackerHitCollectionIterator |
Public Member Functions | |
TrackerHitCollection () | |
~TrackerHitCollection () | |
void | clear () override final |
TrackerHitCollection * | operator-> () |
operator to allow pointer like calling of members a la LCIO More... | |
TrackerHit | create () |
Append a new object to the collection, and return this object. More... | |
template<typename... Args> | |
TrackerHit | create (Args &&... args) |
Append a new object to the collection, and return this object. More... | |
size_t | size () const override final |
number of elements in the collection More... | |
std::string | getValueTypeName () const override |
fully qualified type name of elements - with namespace More... | |
const TrackerHit | operator[] (unsigned int index) const |
Returns the const object of given index. More... | |
TrackerHit | operator[] (unsigned int index) |
Returns the object of a given index. More... | |
const TrackerHit | at (unsigned int index) const |
Returns the const object of given index. More... | |
TrackerHit | at (unsigned int index) |
Returns the object of given index. More... | |
void | push_back (ConstTrackerHit object) |
Append object to the collection. More... | |
void | prepareForWrite () override final |
void | prepareAfterRead () override final |
void | setBuffer (void *address) override final |
bool | setReferences (const podio::ICollectionProvider *collectionProvider) override final |
podio::CollRefCollection * | referenceCollections () override final |
podio::VectorMembersInfo * | vectorMembers () override |
void | setID (unsigned ID) override final |
unsigned | getID () const override final |
bool | isValid () const override final |
const const_iterator | begin () const |
const const_iterator | end () const |
void * | getBufferAddress () override final |
returns the address of the pointer to the data buffer More... | |
std::vector< TrackerHitData > * | _getBuffer () |
Returns the pointer to the data buffer. More... | |
template<size_t arraysize> | |
const std::array< long long, arraysize > | cellID () const |
template<size_t arraysize> | |
const std::array< long, arraysize > | flag () const |
template<size_t arraysize> | |
const std::array< long, arraysize > | g4ID () const |
template<size_t arraysize> | |
const std::array< dd4pod::FourVector, arraysize > | position () const |
template<size_t arraysize> | |
const std::array< dd4pod::FourVector, arraysize > | momentum () const |
template<size_t arraysize> | |
const std::array< double, arraysize > | length () const |
template<size_t arraysize> | |
const std::array< dd4pod::MonteCarloContrib, arraysize > | truth () const |
template<size_t arraysize> | |
const std::array< double, arraysize > | energyDeposit () const |
Detailed Description
A Collection is identified by an ID.
Definition at line 50 of file TrackerHitCollection.h.
Member Typedef Documentation
◆ const_iterator
Definition at line 53 of file TrackerHitCollection.h.
Constructor & Destructor Documentation
◆ TrackerHitCollection()
dd4pod::TrackerHitCollection::TrackerHitCollection | ( | ) |
Definition at line 13 of file TrackerHitCollection.cc.
◆ ~TrackerHitCollection()
dd4pod::TrackerHitCollection::~TrackerHitCollection | ( | ) |
Definition at line 18 of file TrackerHitCollection.cc.
Member Function Documentation
◆ _getBuffer()
|
inline |
Returns the pointer to the data buffer.
Definition at line 128 of file TrackerHitCollection.h.
◆ at() [1/2]
TrackerHit dd4pod::TrackerHitCollection::at | ( | unsigned int | index | ) |
Returns the object of given index.
Definition at line 35 of file TrackerHitCollection.cc.
◆ at() [2/2]
const TrackerHit dd4pod::TrackerHitCollection::at | ( | unsigned int | index | ) | const |
Returns the const object of given index.
Definition at line 27 of file TrackerHitCollection.cc.
◆ begin()
|
inline |
Definition at line 117 of file TrackerHitCollection.h.
◆ cellID()
const std::array< long long, arraysize > dd4pod::TrackerHitCollection::cellID |
Definition at line 173 of file TrackerHitCollection.h.
◆ clear()
|
finaloverride |
Definition at line 50 of file TrackerHitCollection.cc.
◆ create() [1/2]
TrackerHit dd4pod::TrackerHitCollection::create | ( | ) |
Append a new object to the collection, and return this object.
Definition at line 43 of file TrackerHitCollection.cc.
◆ create() [2/2]
TrackerHit dd4pod::TrackerHitCollection::create | ( | Args &&... | args | ) |
Append a new object to the collection, and return this object.
Initialized with the parameters given
Definition at line 165 of file TrackerHitCollection.h.
◆ end()
|
inline |
Definition at line 120 of file TrackerHitCollection.h.
◆ energyDeposit()
const std::array< double, arraysize > dd4pod::TrackerHitCollection::energyDeposit |
Definition at line 243 of file TrackerHitCollection.h.
◆ flag()
const std::array< long, arraysize > dd4pod::TrackerHitCollection::flag |
Definition at line 183 of file TrackerHitCollection.h.
◆ g4ID()
const std::array< long, arraysize > dd4pod::TrackerHitCollection::g4ID |
Definition at line 193 of file TrackerHitCollection.h.
◆ getBufferAddress()
|
inlinefinaloverride |
returns the address of the pointer to the data buffer
Definition at line 125 of file TrackerHitCollection.h.
◆ getID()
|
inlinefinaloverride |
Definition at line 108 of file TrackerHitCollection.h.
◆ getValueTypeName()
|
inlineoverride |
fully qualified type name of elements - with namespace
Definition at line 77 of file TrackerHitCollection.h.
◆ isValid()
|
inlinefinaloverride |
Definition at line 112 of file TrackerHitCollection.h.
◆ length()
const std::array< double, arraysize > dd4pod::TrackerHitCollection::length |
Definition at line 223 of file TrackerHitCollection.h.
◆ momentum()
const std::array< dd4pod::FourVector, arraysize > dd4pod::TrackerHitCollection::momentum |
Definition at line 213 of file TrackerHitCollection.h.
◆ operator->()
|
inline |
operator to allow pointer like calling of members a la LCIO
Definition at line 63 of file TrackerHitCollection.h.
◆ operator[]() [1/2]
TrackerHit dd4pod::TrackerHitCollection::operator[] | ( | unsigned int | index | ) |
Returns the object of a given index.
Definition at line 31 of file TrackerHitCollection.cc.
◆ operator[]() [2/2]
const TrackerHit dd4pod::TrackerHitCollection::operator[] | ( | unsigned int | index | ) | const |
Returns the const object of given index.
Definition at line 23 of file TrackerHitCollection.cc.
◆ position()
const std::array< dd4pod::FourVector, arraysize > dd4pod::TrackerHitCollection::position |
Definition at line 203 of file TrackerHitCollection.h.
◆ prepareAfterRead()
|
finaloverride |
Definition at line 70 of file TrackerHitCollection.cc.
◆ prepareForWrite()
|
finaloverride |
Definition at line 56 of file TrackerHitCollection.cc.
◆ push_back()
void dd4pod::TrackerHitCollection::push_back | ( | ConstTrackerHit | object | ) |
Append object to the collection.
Definition at line 91 of file TrackerHitCollection.cc.
◆ referenceCollections()
|
inlinefinaloverride |
Definition at line 97 of file TrackerHitCollection.h.
◆ setBuffer()
|
finaloverride |
Definition at line 103 of file TrackerHitCollection.cc.
◆ setID()
|
inlinefinaloverride |
Definition at line 101 of file TrackerHitCollection.h.
◆ setReferences()
|
finaloverride |
Definition at line 86 of file TrackerHitCollection.cc.
◆ size()
|
finaloverride |
number of elements in the collection
Definition at line 39 of file TrackerHitCollection.cc.
◆ truth()
const std::array< dd4pod::MonteCarloContrib, arraysize > dd4pod::TrackerHitCollection::truth |
Definition at line 233 of file TrackerHitCollection.h.
◆ vectorMembers()
|
inlineoverride |
Definition at line 99 of file TrackerHitCollection.h.
The documentation for this class was generated from the following files: